Appendix G - Developing Action Builder Plugins
Plugins are used to extend the functionality of Reality Action system. You can add a lot of new functionality by writing your own plugins. These plugins can be written in C#.
The Plugin Structure, Required Files, and Their Locations
The first thing you need to do is to create a new "Class Library" project in the solution. It's a good practice to place all plugins into \Plugins directory in the root of your solution.
A recommended name for a plugin project is "ZDAction.Plugin.{Group}.{Name}".
{Group} is your plugin group (for example, "Network").
{Name} is your plugin name (for example, "Udp").
For example, Udp plugin has the following name: ZDAction.Plugin.Network.Udp.
ZDAction prefix is a requirement. All plugin names start with ZDAction prefix.
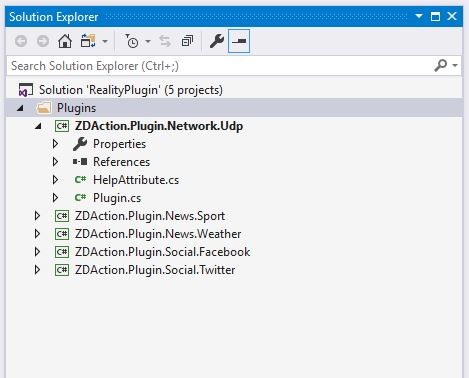
The project structure of Network.Udp plugin looks like the image below:

Plugin project requires two main classes. First one is Plugin, the second one is HelperAttribute.
Plugin Class is the entry point of Plugin.
Reality Action system can use reflection to discover information about plugin methods at run time and to create an instance of a type at runtime.
Plugin class looks like the image below:
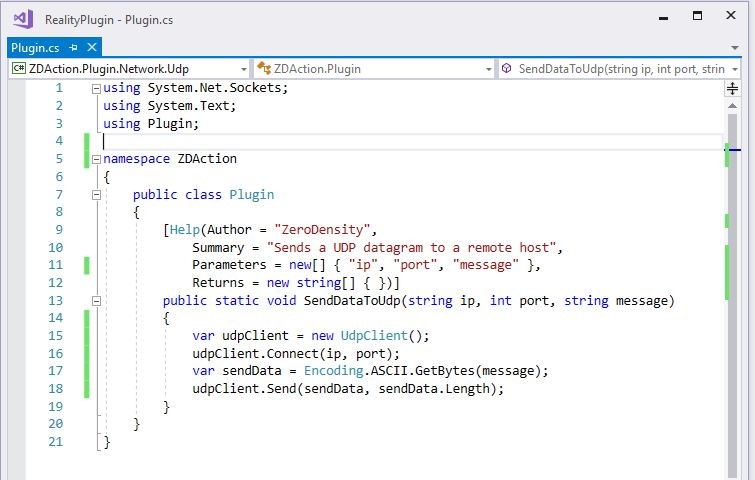
There are 3 importan things:
Plugin Class Namespace must be ZDAction.
The HelpAttribute must be defined(for only public methods)
Plugin Class methods must be static.
Help attribute is used by Reality Action system for retrieving information plugin methods.
The HelpAttribute class looks like the image below:
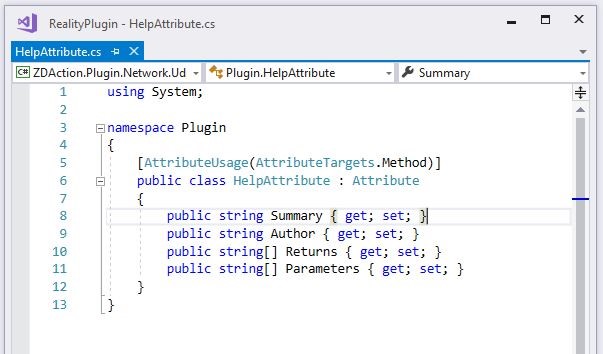
The HelpAttribute class has four public properties for storing information.
Name | Description |
Summary | Summary of method |
Author | Author of method |
Returns | Summary of method return value(s) The return type is the data type of the value the method returns. If the method is not returning any values, then the return type is void. The method return type can be void, tuple or any of the data types defined below |
Parameters | Summary of method input parameter(s) The method input parameter type can be any of the data types defined below |
Plugin system supports below data types for input parameters and returns values
Data Type | Represents |
Boolean | Boolean value |
Color * | Color value |
Float | Float value |
Integer | Integer value |
Rotator * | Rotator value |
Size * | Size value |
String | String value |
Texture(deprecated) * | Texture value |
Transform * | Transform value |
Vector * | Vector value |
Vector2D * | Vector2D value |
These data types are derived from the ZDCore.dll. In order to use this data types in your plugin, you must first add a reference to it.
Sample plugin method looks like the image below:
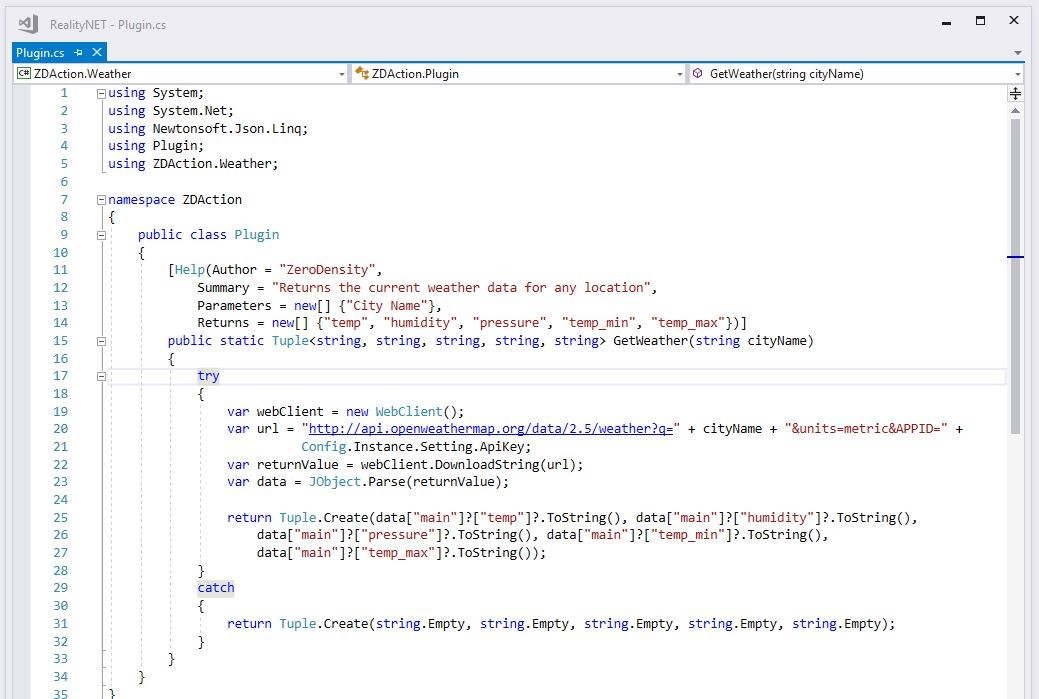